Qt Signal Slot Thread Context
Introduction
Qt::AutoConnection: If the receiver lives in the thread that emits the signal, Qt::DirectConnection is used. Otherwise, Qt::QueuedConnection is used. The connection type is determined when the signal is emitted. Qt::DirectConnection: This slot is invoked immediately when the signal is emitted. The slot is executed in the signaling thread.
Remember old X-Windows call-back system? Generally it isn't type safe and flexible. There are many problems with them. Qt offers a new event handling system: signal-slot connections. Imagine an alarm clock. When alarm is ringing, a signal is being sent (emit). And you're handling it in a slot.

- Every QObject class may have as many signals and slots as you want
- You can emit signals only from within that class, where the signal is located
- You can connect signal with another signal (make chains of signals);
- Every signal and slot can have unlimited count of connections with other.
- ATTENTION! You can't set default value in slot attributes e.g. void mySlot(int i = 0);
- This wrapper provides the signals, slots and methods to easily use the thread object within a Qt project. To use it, prepare a QObject subclass with all your desired functionality in it. Then create a new QThread instance, push the QObject onto it using moveToThread(QThread.) of the QObject instance and call start on the QThread instance.
- QtCore.SIGNAL and QtCore.SLOT macros allow Python to interface with Qt signal and slot delivery mechanisms. This is the old way of using signals and slots. The example below uses the well known clicked signal from a QPushButton. The connect method has a non python-friendly syntax. It is necessary to inform the object, its signal (via macro.
- Qt uses signals and slots normally in a single thread, so calling a signal will call a slot in the same thread signal called. Is it any way to use a signal-slot mechanism to pass a message to qt thread (so slot will be called later in specified thread's context)?
Connection
You can connect signal with this template:
QObject::connect (
);
You have to wrap const char * signal and const char * method into SIGNAL() and SLOT() macros.
And you also can disconnect signal-slot:
QObject::disconnect (
);
Deeper
Widgets emit signals when events occur. For example, a button will emit a clicked signal when it is clicked. A developer can choose to connect to a signal by creating a function (a slot) and calling the connect() function to relate the signal to the slot. Qt's signals and slots mechanism does not require classes to have knowledge of each other, which makes it much easier to develop highly reusable classes. Since signals and slots are type-safe, type errors are reported as warnings and do not cause crashes to occur.
For example, if a Quit button's clicked() signal is connected to the application's quit() slot, a user's click on Quit makes the application terminate. In code, this is written as
Qt Signal Slot Thread Contexto
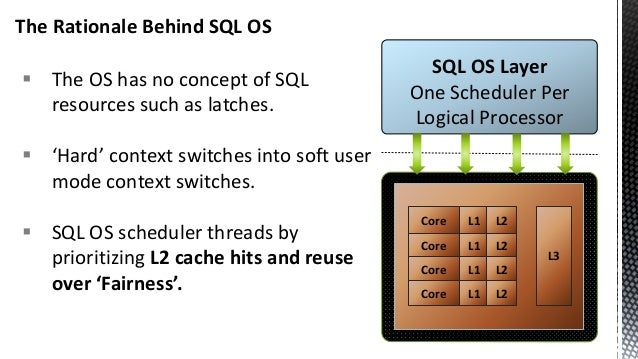
connect(button, SIGNAL (clicked()), qApp, SLOT (quit()));
Connections can be added or removed at any time during the execution of a Qt application, they can be set up so that they are executed when a signal is emitted or queued for later execution, and they can be made between objects in different threads.

The signals and slots mechanism is implemented in standard C++. The implementation uses the C++ preprocessor and moc, the Meta Object Compiler, included with Qt. Code generation is performed automatically by Qt's build system. Developers never have to edit or even look at the generated code.
In addition to handling signals and slots, the Meta Object Compiler supports Qt's translation mechanism, its property system, and its extended runtime type information. It also makes runtime introspection of C++ programs possible in a way that works on all supported platforms.
To make moc compile the meta object classes don't forget to add the Q_OBJECT macro to your class.
Qt - Passing custom objects among threads
- Details
- Category: Programming
- Written by Nandan Banerjee
- Hits: 13035
Communication between threads in a qt program is essentially done by using signals/slots. This is by far one of the most easiest and stable mode of communication amongst threads of a program.
For example, let us suppose that one thread needs to send an integer value to another thread. All the programmer needs to do is simply create a dispatch signal with two arguments, the thread id and the integer value. Thus, it can be achieved by this simple line –
Similarly, a receive slot with the same arguments needs to be created to receive the signal.
Now, this will work very nicely when one is dealing with the predefined primitive or the qt data types. This is because they are already registered and hence it is not a problem for qt to recognise those data types.
The problem arises when one wants to pass a custom data type (any class or structure that has a public default constructor, a public copy constructor, and a public destructor can be registered). Then, the user defined class or a class defined in a library not part of qt can be passed using signals/slots after registering.

The qRegisterMetaType() function is used to make the type available to non-template based functions, like the queued signal and slot connections.
This is done in the following way –
where name can be any custom data type.
For example, let us take a program which will capture the image from the webcam and display it in a QLabel on the GUI. To achieve this, two approaches can be taken. Run the camera grabbing function in the main UI thread or in a different thread and reducing the work of the UI thread significantly. If it is run in the main UI thread, then the chances of the UI thread not responding is very high. Therefore, it is always desirable make a separate thread and use it instead to run the camera grabbing function.
In this tutorial, we will use the openCV library to grab an image from the webcam and use the signal/slot mechanism to send the image (IplImage type) to the UI thread.
After creating a new qtGUI project, a new class is created (say “webcamThread”) with QThread as its parent class. A run() function is defined and a new signal with the image as the argument is defined.
In the MainWindow file, a slot is defined to handle the signal from the webcamThread. This image is then converted to the QImage format and then displayed in the QLabel. So, a smooth and pleasant webcam feed can be achieved using this.
Qt code for the webcam feed -
The openCV library needs to be present in the system and the paths should be appropriately set.
// webcamthread.h
// webcamthread.cpp
Qt Signals And Slots Tutorial
Now the code for the MainWindow. The signals are connected with the slots and the event handlers are defined.
// mainwindow.h
// mainwindow.cpp
Qt Signal Slot Example
In the UI editor, two buttons (Start and Stop) and a label of size 320 by 240 need to be created. Then, just compile and run. So, it can be seen that objects of the class “IplImage” from the openCV library can be easily passed between the threads just by registering the class.